Localization
The cart can be translated in any language. Our language files are open source on GitHub.
We maintain the en translation. If you want to submit a new translation, use the en one as a baseline.
Each language file is a JSON document.
Default behavior
The cart will always try to load the right language file based on the lang
attribute in the <html>
tag of your site:
<!DOCTYPE html>
<html lang="fr">
<head>
<title></title>
</head>
<body>
</body>
</html>
In the example above, the cart will try to load the fr.json
language file. If it exists, the cart will be displayed in French. We automatically fallback to English.
Usage in theme
In any overridable component of the cart theme, you have access to a method named $localize
. This method takes one parameter: the locale key.
For instance:
Language file en.json
:
{
"actions": {
"continue_shopping": "Continue shopping",
...
},
...
}
Overridable component:
<h1>{{ $localize('actions.continue_shopping') }}</h1>
Output:
<h1>Continue shopping</h1>
Using our JavaScript API
You can also set the cart language using our JavaScript API.
This is possible via the session
API:
<script>
document.addEventListener('snipcart.ready', function() {
Snipcart.api.session.setLanguage('fr');
});
</script>
The example above will set the cart language to French.
You can also change labels with the JavaScript API. Let's say you want to change Continue shopping to Go back to store:
<script>
document.addEventListener('snipcart.ready', function() {
Snipcart.api.session.setLanguage('en', {
actions: {
continue_shopping: "Go back to store"
}
});
});
</script>
This will only replace this specific label. It can come in handy when fine-tuning your Snipcart integration.
You can also use the JavaScript API to register extra locales. This will allow your translation to cover new labels you have added to our default theme, for instance.
How to contribute
To submit a new translation, you'll need to open a pull request. To do so, start by forking our repository. Open the repository on GitHub, then hit the Fork button. This will create a copy of the repository under your GitHub account.
Once the repository is forked, clone it on your computer. Open the project with your favorite code editor. You’ll see a folder named locales
—it contains the language files.
A language file is a JSON document that contains all the labels used throughout the cart. To add a new language, create a new file in this folder. The file should be named with the locale ISO code—for instance en.json
for English, or fr.json
for French.
Translate all the values in this file, and then commit and push your changes to your repository. Then, you will be able to open a pull request. On our side, we'll review the pull request and merge it if everything looks fine.
Please refer to this article about how forks and pull requests work on GitHub.
Regional locales
Depending on where you're located, wording might change. For instance, some English words and expressions are different in the UK than in the US, so you might want to use a regional location file, like en-GB
, for instance.
When you create a regional locale, you can override only necessary locales, you don't need to rewrite the whole file. Our building process will make sure to merge everything together. Here's an example of what we did for the fr-FR
regional locale:
{
"actions": {
"continue_shopping": "Continuer les achats"
}
}
Payment methods
We're often asked about how to change payment method names in the checkout form. It's possible to use our JavaScript API to do this quickly.
All payment method names are localized, in payment.methods
object (see en.json
on Github).
Let's say you want to change Pay later to Pay at delivery. You could do this:
<script>
document.addEventListener('snipcart.ready', function() {
Snipcart.api.session.setLanguage('en', {
"payment": {
"methods": {
"deferred_payment": "Pay at delivery"
}
}
});
});
</script>
Shipping methods
Introduced in v3.3.3, custom shipping methods can now be localized. Specify the localization id in the merchant dashboard when creating a new shipping method, then you can set your translations using our localization API.
<script>
document.addEventListener('snipcart.ready', function() {
Snipcart.api.session.setLanguage('en', {
"shipping": {
"methods": {
"localization-id": "Localized shipping method"
}
}
});
});
</script>
Quick recipes
The following examples show ways to customize the checkout using our Localization API.
Deferred payments
Our deferred payments feature allows merchants to let their customers place orders without payment, which means that the merchant will then be responsible to collect the money somehow. It might be important to display accurate information on how this payment process will occur directly in the checkout form.
You can override these locales: payment.form.deferred_payment_title
and deferred_payment_instructions
to do so.
By default, the checkout form looks like this:

Let's say you want to change Deferred Payment by Pay at delivery and include details on how it will work.
<script>
document.addEventListener('snipcart.ready', function() {
Snipcart.api.session.setLanguage('en', {
"payment": {
"form": {
"deferred_payment_title": "Pay at delivery",
"deferred_payment_instructions": "Payment will be made with a credit card at the delivery of your order."
}
}
});
});
</script>
Checkout form will then look like this:
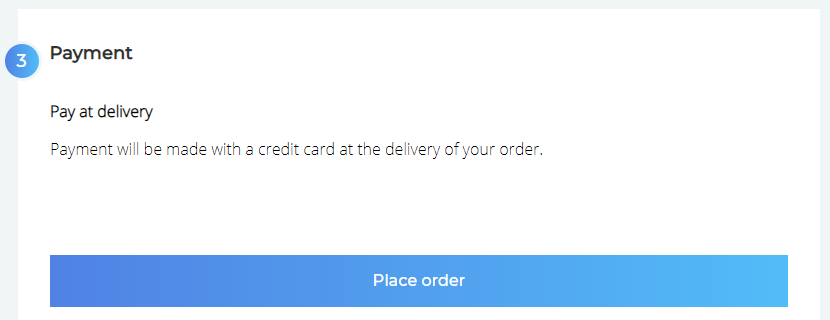